Discovering open source WPF components - Orc.Search
In the discovering open source WPF components series, I will look into useful open source components that can be used to create WPF apps. This weeks component is Orc.Search.
Most applications sooner or later need a search functionality. Since most .net applications work with models (objects containing the actual information users want to search for), it might be hard to implement such functionality.
A great open-source search engine for indexing and searching is Lucene.net, but it requires quite some work to implement it from scratch in an application. Orc.Search provides a great wrapper around Lucene.net in 3 simple steps. Of course it also comes with xaml controls to easily add search boxes with status indicators to your applications.
Step 1: decorate your models with the right attributes
public class Person
{
[SearchableProperty(SearchName = "firstname")]
public string FirstName { get; set; }
[SearchableProperty(SearchName = "lastname")]
public string LastName { get; set; }
public int Age { get; set; }
}
An alternative to using attributes is to implement a custom IMetadataProvider (you are never forced to only use attributes).
Step 2: Add the projects to the search service
searchService.AddObjects(persons.Select(x => new ReflectionSearchable(x));
Step 3: Use the Search method
Once the objects are indexed, you can easily use the search method to search for any values:
var results = searchService.Search("my query");
Enjoy your results!
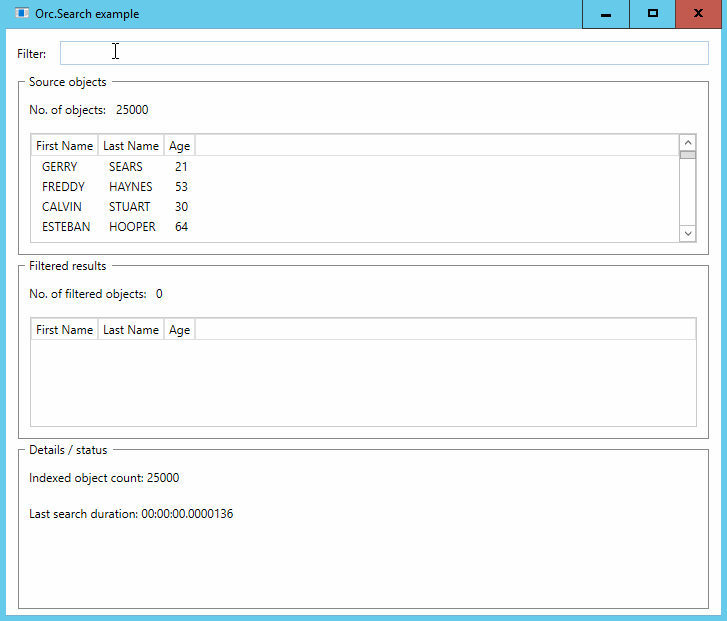